Option 2 : Cookie Restore
How does this solution work?
Addingwell’s Cookie Restore solution involves the implementation of a “Master Cookie,” which is defined by your navigation domain’s server and is unique for each user. (More details about this Master Cookie can be found in the Detailed Implementation section).
During the First 7 Days
When a user visits your navigation domain within the first 7 days, Marketing cookies are still present in the user’s browser. Therefore, there is no need to restore these cookies, as they are well-defined. The Master Cookie is placed by your navigation domain’s server and remains valid for 13 months. On Addingwell’s side, the Master Cookie defined for this user by your server will be associated with the Marketing cookies and stored in a database. This “Master Cookie + Marketing cookies” association is preserved for future use.
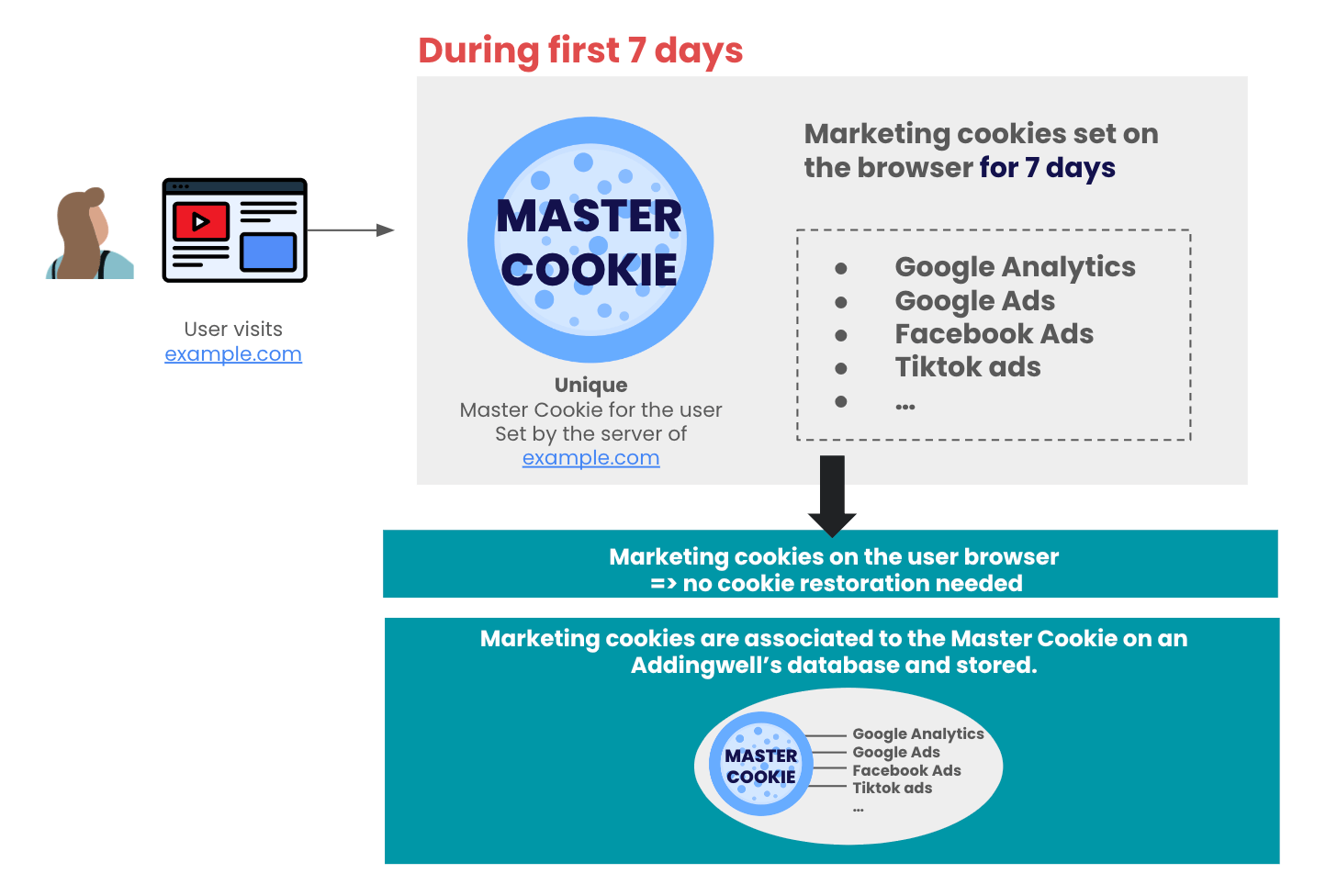
Beyond 7 Days
After 7 days, when the user revisits your website, the Marketing cookies are no longer present in their browser. However, the Master Cookie remains, as it is defined by your navigation server and has a 13-month validity. Addingwell’s database is queried, and the “Master Cookie + Marketing cookies” association is retrieved. The Cookie Restore solution then pushes the Marketing cookies associated with the user’s Master Cookie back into their browser!
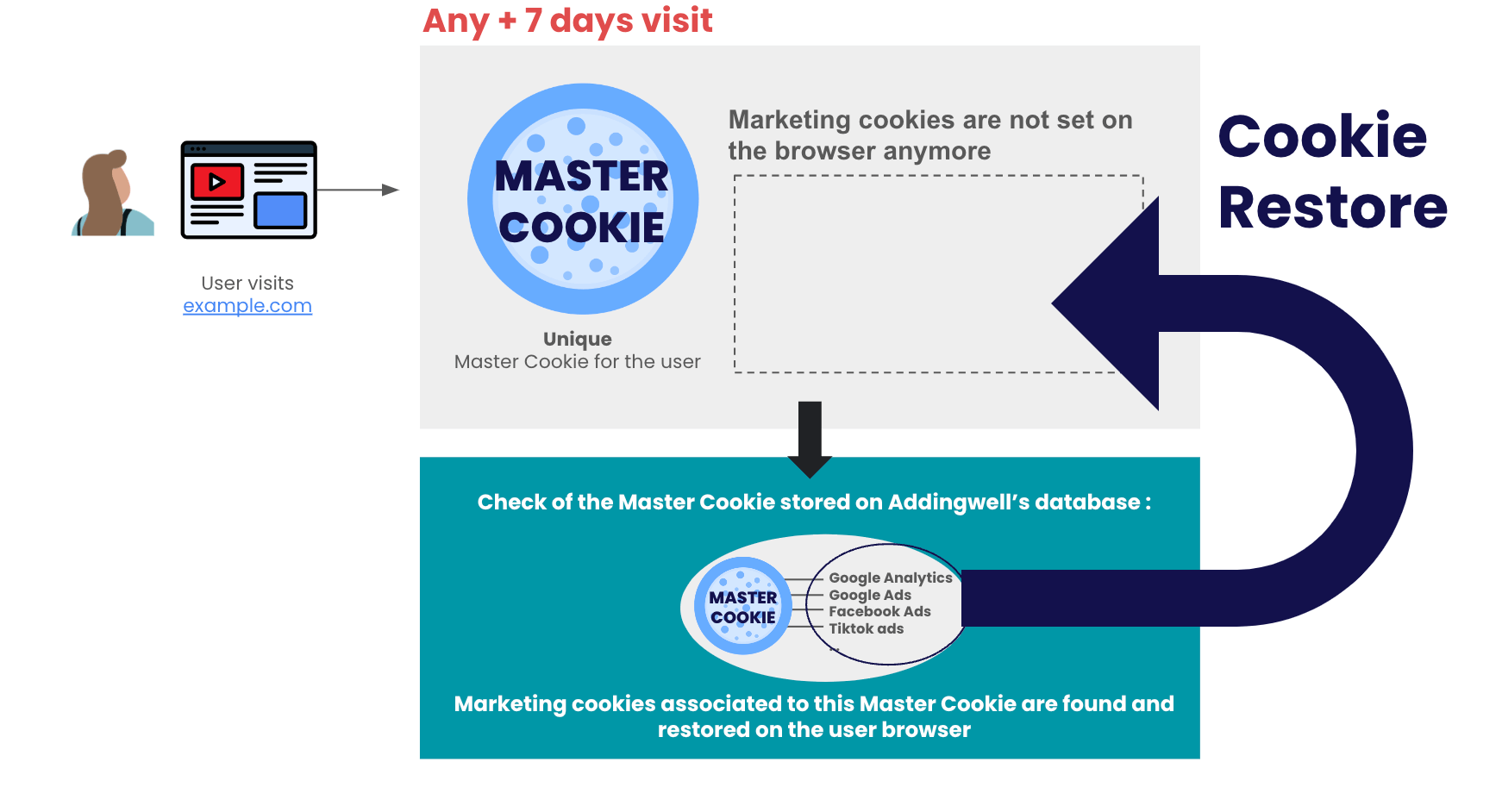
For this option, it is necessary to have a “new generation” first-party cookie that is unique per user. This cookie must be set by the server hosting the entire website to be considered valid.
Implementation of the “Master Cookie”
Technical Specifications
To be compliant, this cookie must meet the following specifications:
- Lifespan: The cookie’s lifespan must be sufficiently long. It should preferably be longer than the lifespan of marketing cookies. However, it should not exceed 13 months to comply with the General Data Protection Regulation (GDPR).
- Accessibility: The cookie must be accessible across the entire site domain and all its subdomains. Example: the cookie should be set on
.mysite.com
rather thanmysite.com
. - Origin: The cookie must be set by the server of the site. It should not be set by client-side executed JavaScript code.
- Persistence: The cookie must be available on all pages of the site.
- Stability: The cookie value must be stable and should not change over time.
- Uniqueness: The cookie value must be unique per visitor.
Example of a “Master Cookie”
Below is an example of a cookie set by the mysite.com server with a lifespan of 13 months.
This cookie is accessible on all pages of the site and all its subdomains.
The cookie’s name is _aw_master_id
, and its value is a unique visitor identifier 646035e9-503e-412e-8b99-5ee47560f882
.
Name | Value | Domain | Path | Expires / Max-Age | HttpOnly | Secure | SameSite |
---|---|---|---|---|---|---|---|
_aw_master_id | 646035e9-503e-412e-8b99-5ee47560f882 | .mysite.com | / | 2025-09-02T13:33:00.341Z | true | true | Lax |
Implementation Help
To simplify the installation of this cookie, you can use the following examples depending on the server language or CMS (Shopify, WordPress, etc.).
If your site is not compatible with the list of examples below, please contact Addingwell support.
Custom (PHP)
Here is an example of code to create or update a cookie that meets the technical specifications on a PHP server.
function setMasterCookie() {
$cookieName = '_aw_master_id';
$cookieLifetime = time() + (60 * 60 * 24 * 30 * 13);
$cookieDomain = getMainDomain($_SERVER['HTTP_HOST']);
if (!isset($_COOKIE[$cookieName])) {
$cookieValue = $this->generateUUID();
} else {
$cookieValue = $_COOKIE[$cookieName];
}
setcookie(
$cookieName,
$cookieValue,
$cookieLifetime,
'/',
$cookieDomain,
true,
true
);
}
function generateUUID() {
return sprintf(
'%04x%04x-%04x-%04x-%04x-%04x%04x%04x',
mt_rand(0, 0xFFFF), mt_rand(0, 0xFFFF),
mt_rand(0, 0xFFFF),
mt_rand(0, 0x0FFF) | 0x4000,
mt_rand(0, 0x3FFF) | 0x8000,
mt_rand(0, 0xFFFF), mt_rand(0, 0xFFFF), mt_rand(0, 0xFFFF)
);
}
function getMainDomain($url) {
$composedTlds = [
'co.uk', 'gov.uk', 'ac.uk', 'org.uk', 'net.uk', 'sch.uk', 'nhs.uk', 'police.uk',
'com.au', 'net.au', 'org.au', 'edu.au', 'gov.au', 'asn.au', 'id.au',
'co.jp', 'ac.jp', 'ne.jp', 'or.jp', 'go.jp', 'ed.jp', 'ad.jp', 'gr.jp',
'com.cn', 'net.cn', 'gov.cn', 'org.cn', 'edu.cn', 'mil.cn', 'ac.cn',
'com.br', 'net.br', 'org.br', 'gov.br', 'edu.br', 'mil.br', 'art.br', 'coop.br',
'co.in', 'net.in', 'org.in', 'gov.in', 'ac.in', 'res.in', 'edu.in', 'mil.in', 'nic.in',
'gc.ca', 'gov.ca',
'com.de', 'net.de', 'org.de',
'gov.it', 'edu.it',
'asso.fr', 'nom.fr', 'prd.fr', 'presse.fr', 'tm.fr', 'com.fr', 'gouv.fr',
'com.es', 'nom.es', 'org.es', 'gob.es', 'edu.es',
'co.za', 'net.za', 'gov.za', 'org.za', 'edu.za',
'com.mx', 'net.mx', 'org.mx', 'edu.mx', 'gob.mx',
'com.ru', 'net.ru', 'org.ru', 'edu.ru', 'gov.ru',
'co.kr', 'ne.kr', 'or.kr', 're.kr', 'pe.kr', 'go.kr', 'mil.kr',
'com.sg', 'net.sg', 'org.sg', 'edu.sg', 'gov.sg', 'per.sg',
'com.my', 'net.my', 'org.my', 'gov.my', 'edu.my', 'mil.my',
'com.hk', 'net.hk', 'org.hk', 'gov.hk', 'edu.hk', 'idv.hk',
'com.ar', 'net.ar', 'org.ar', 'gov.ar', 'edu.ar', 'int.ar',
'com.tr', 'net.tr', 'org.tr', 'gov.tr', 'edu.tr', 'mil.tr',
];
// Remove protocol if present
$domain = preg_replace('/^https?:\/\//', '', $url);
// Remove www. if present
$domain = preg_replace('/^www\./', '', $domain);
// Split the domain into parts
$parts = explode('.', $domain);
// Check against composed TLDs
for ($i = 0; $i < count($parts) - 1; $i++) {
$possibleTld = implode('.', array_slice($parts, $i));
if (in_array($possibleTld, $composedTlds)) {
$main_domain = implode('.', array_slice($parts, $i - 1));
return $main_domain;
}
}
// Default to last two parts if no composed TLD matches
return implode('.', array_slice($parts, -2));
}
setMasterCookie();
A _aw_master_id
cookie should appear on the first page load without any additional actions on the page.
The lifespan of this cookie is 13 months (390 days).
For the next step, please specify _aw_master_id
as the cookie name.
Enabling Cookie Restore
From your Addingwell Container, click on Cookie monitoring in the left menu, then on Cookie Restore.
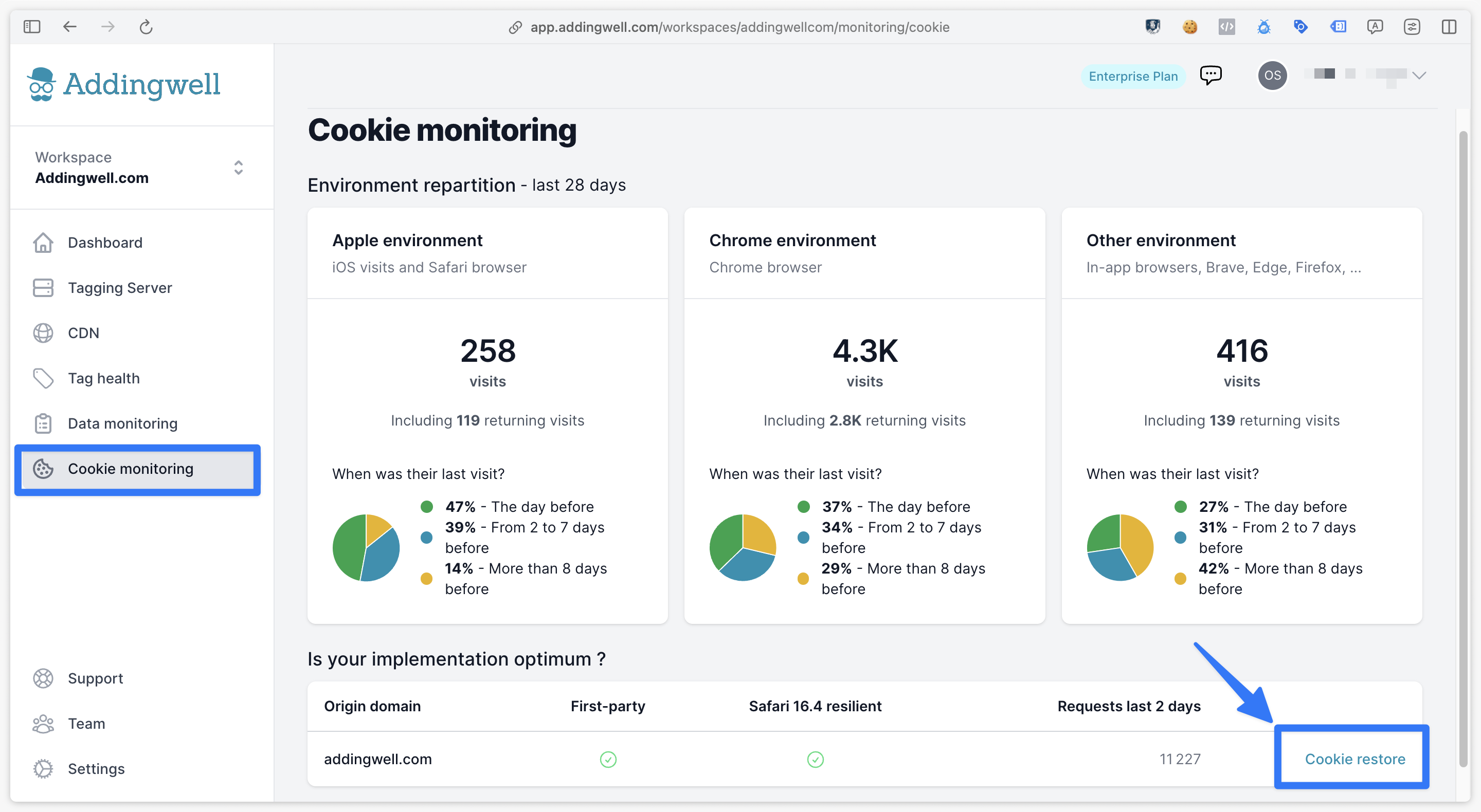
Fill in the form fields.
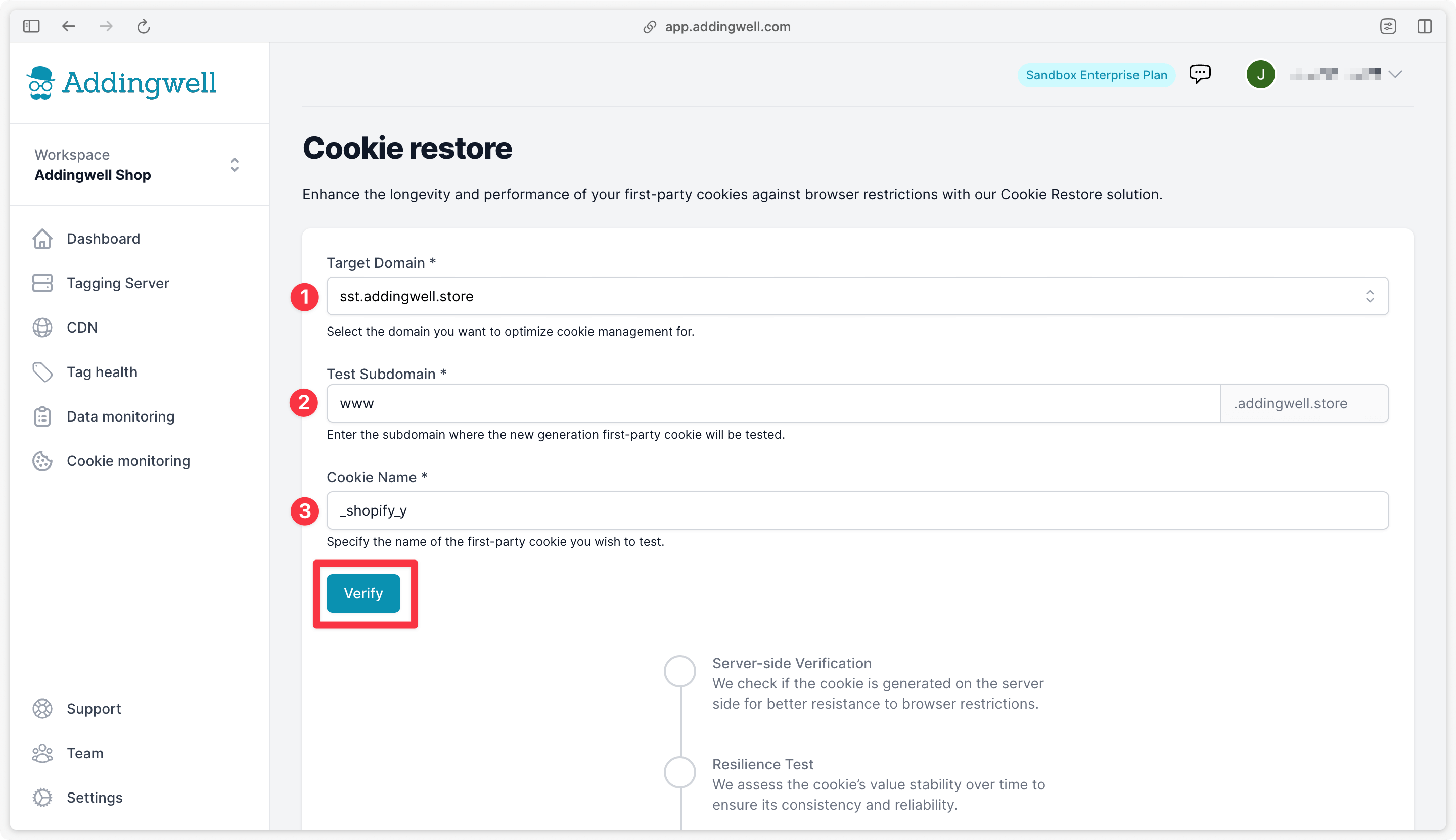
Target Domain
Enter the subdomain or domain of the site where you want to test the cookie.
Domain to test
Enter the subdomain or domain of the site where you want to test the cookie.
Nom du cookie
Enter the name of the “Master Cookie”.
Enable Cookie Restore
Once everything is filled out, you can click on Verify.
The verification of your “Master Cookie” is done automatically and may take a few minutes.
Select the zone if it is not already done, then click on “Enable”.
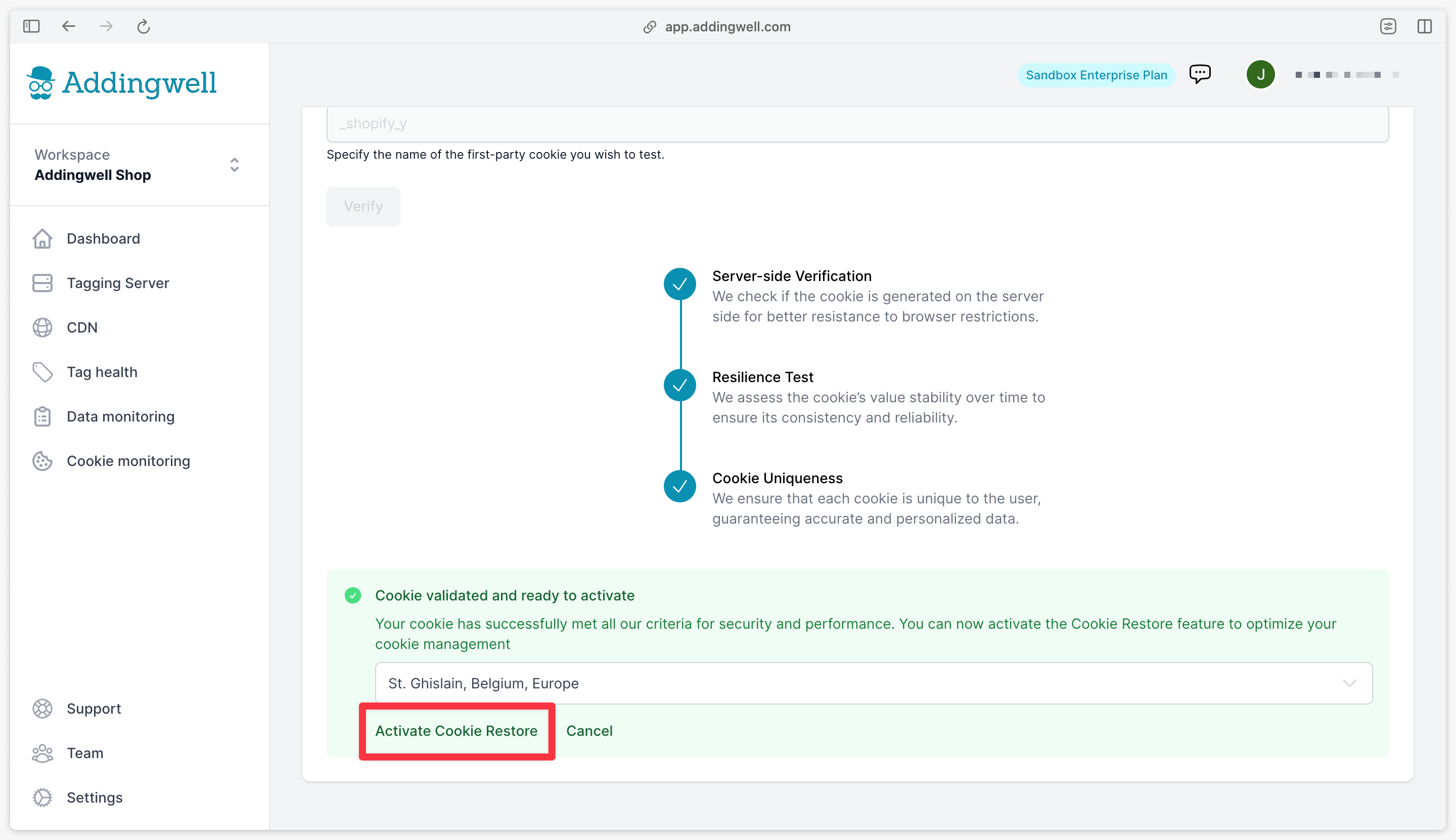
You have now completed the implementation of the “Cookie Restore” feature. You can proceed to the next step: Check the results.